How To Add Dictionary To Array In Swift
Equally discussed in the introduction to this section, collections are flexible "containers" that let yous store any number of values together. Before discussing these collections, yous need to understand the concept of mutable vs immutable collections.
As part of exploring the differences between the collection types, you'll too consider performance: how speedily the collections can perform certain operations, such as calculation to the collection or searching through it.
The usual fashion to talk about operation is with big-O annotation. If you're not familiar with it already, outset reading the side by side chapter for a brief introduction.
Big-O notation is a way to depict running time, or how long an operation takes to complete. The idea is that the exact time an operation takes isn't of import; it's the relative difference in scale that matters.
Imagine you accept a listing of names in some random society, and yous have to wait up the showtime name on the list. Information technology doesn't matter whether the list has a single proper noun or a million names — glancing at the very first name always takes the aforementioned amount of time. That'south an example of a constant time operation, or O(1) in big-O notation.
At present say you take to find a particular name on the list. Y'all demand to browse through the list and wait at every single proper noun until you either observe a match or achieve the end. Once again, nosotros're not concerned with the verbal corporeality of fourth dimension this takes, just the relative time compared to other operations.
To effigy out the running fourth dimension, think in terms of units of work. You need to look at every proper name, so consider at that place to exist one "unit" of work per name. If you had 100 names, that'south 100 units of work. What if you double the number of names to 200? How does that change the amount of work?
The answer is it too doubles the amount of work. Similarly, if you quadruple the number of names, that quadruples the amount of work.
This increase in piece of work is an example of a linear fourth dimension operation, or O(Northward) in big-O notation. The input size is the variable N, which ways the amount of time the process takes is also Northward. At that place's a directly, linear relationship between the input size (the number of names in the list) and the time information technology will take to search for ane name.
You can see why constant time operations employ the number one in O(1). They're just a single unit of work, no thing what!
Y'all can read more about big-O note by searching the Web. You'll only demand constant time and linear time in this book, but there are other such fourth dimension complexities out there.
Large-O note is particularly important when dealing with collection types because collections can shop vast amounts of information. Yous need to be aware of running times when you add, delete or edit values.
For case, if collection type A has constant-time searching and drove blazon B has linear-time searching, which you choose to employ will depend on how much searching yous're planning to do.
Mutable versus immutable collections
Just like the previous types you've read nigh, such every bit Int
or String
, when y'all create a collection you lot must declare information technology every bit either a constant or a variable.
If the drove doesn't demand to change after you lot've created information technology, you should get in immutable by declaring it as a constant with let
. Alternatively, if you demand to add, remove or update values in the drove, and then you should create a mutable collection by declaring it as a variable with var
.
Arrays
Arrays are the most mutual collection type you lot'll run into in Swift. Arrays are typed, just like regular variables and constants, and shop multiple values like a simple list.
What is an array?
An array is an ordered collection of values of the same type. The elements in the array are zero-indexed, which ways the index of the start element is 0, the index of the second chemical element is 1, and then on. Knowing this, yous tin work out that the final element'due south index is the number of values in the assortment minus one.
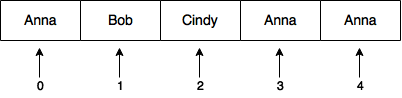
When are arrays useful?
Arrays are useful when you want to store your items in a item order. Yous may want the elements sorted, or yous may need to fetch elements by index without iterating through the entire array.
Creating arrays
The easiest way to create an array is by using an array literal. This is a concise way to provide array values. An assortment literal is a list of values separated by commas and surrounded by foursquare brackets.
let evenNumbers = [2, 4, 6, viii]
var subscribers: [String] = []
permit allZeros = Array(repeating: 0, count: 5) // [0, 0, 0, 0, 0]
let vowels = ["A", "E", "I", "O", "U"]
Accessing elements
Being able to create arrays is useless unless you know how to fetch values from an array. In this department, you'll learn several unlike ways to admission elements in an array.
Using backdrop and methods
Imagine you're creating a game of cards, and y'all desire to store the players' names in an assortment. The list will need to modify equally players join or exit the game, then you lot demand to declare a mutable array:
var players = ["Alice", "Bob", "Cindy", "Dan"]
impress(players.isEmpty) // > imitation
if players.count < 2 { print("Nosotros demand at to the lowest degree ii players!") } else { print("Let's start!") } // > Let'southward start!
var currentPlayer = players.first
print(currentPlayer as Any) // > Optional("Alice")
print(players.terminal as Whatever) // > Optional("Dan")
currentPlayer = players.min() impress(currentPlayer equally Any) // > Optional("Alice")
print([2, iii, 1].first as Any) // > Optional(ii) print([2, iii, one].min() every bit Any) // > Optional(ane)
if let currentPlayer = currentPlayer { print("\(currentPlayer) volition start") } // > Alice will get-go
Using subscripting
The most convenient way to access elements in an array is past using the subscript syntax. This syntax lets you access any value directly by using its index inside square brackets:
var firstPlayer = players[0] print("First role player is \(firstPlayer)") // > Offset player is "Alice"
var player = players[4] // > fatal error: Index out of range
Using countable ranges to make an ArraySlice
You lot can use the subscript syntax with countable ranges to fetch more than than a single value from an array. For example, if y'all'd like to become the side by side two players, you could exercise this:
let upcomingPlayersSlice = players[1...2] print(upcomingPlayersSlice[i], upcomingPlayersSlice[2]) // > "Bob Cindy\n"
let upcomingPlayersArray = Assortment(players[ane...ii]) print(upcomingPlayersArray[0], upcomingPlayersArray[1]) // > "Bob Cindy\due north"
Checking for an element
You can check if there's at least one occurrence of a specific element in an array by using contains(_:)
, which returns true
if it finds the element in the array, and imitation
otherwise.
func isEliminated(player: String) -> Bool { !players.contains(player) }
print(isEliminated(player: "Bob")) // > false
players[ane...iii].contains("Bob") // true
Modifying arrays
You can brand all kinds of changes to mutable arrays, such as calculation and removing elements, updating existing values, and moving elements around into a different social club. In this section, you'll see how to work with the array to friction match up what'south going on with your game.
Appending elements
If new players desire to join the game, they need to sign upward and add their names to the assortment. Eli is the commencement role player to join the existing four players. You can add Eli to the end of the array using the append(_:)
method:
players.append("Eli")
players += ["Gina"]
impress(players) // > ["Alice", "Bob", "Cindy", "Dan", "Eli", "Gina"]
Inserting elements
An unwritten dominion of this carte du jour game is that the players' names take to exist in alphabetical order. This listing is missing a role player that starts with the letter of the alphabet F. Luckily, Frank has just arrived. You want to add him to the list between Eli and Gina. To do that, you can use the insert(_:at:)
method:
players.insert("Frank", at: 5)
Removing elements
During the game, the other players caught Cindy and Gina cheating. They should be removed from the game! You know that Gina is last in the players list, so you can remove her hands with the removeLast()
method:
var removedPlayer = players.removeLast() impress("\(removedPlayer) was removed") // > Gina was removed
removedPlayer = players.remove(at: 2) impress("\(removedPlayer) was removed") // > Cindy was removed
Mini-practise
Employ firstIndex(of:)
to determine the position of the chemical element "Dan"
in players
.
Updating elements
Frank has decided anybody should telephone call him Franklin from now on. Y'all could remove the value "Frank"
from the array and so add together "Franklin"
, but that'south too much work for a elementary chore. Instead, you should utilize the subscript syntax to update the proper name.
impress(players) // > ["Alice", "Bob", "Dan", "Eli", "Frank"] players[4] = "Franklin" print(players) // > ["Alice", "Bob", "Dan", "Eli", "Franklin"]
players[0...1] = ["Donna", "Craig", "Brian", "Anna"] print(players) // > ["Donna", "Craig", "Brian", "Anna", "Dan", "Eli", "Franklin"]
Moving elements
Accept a look at this mess! The players
array contains names that start with A to F, just they aren't in the right gild, which violates the rules of the game.
let playerAnna = players.remove(at: 3) players.insert(playerAnna, at: 0) print(players) // > ["Anna", "Donna", "Craig", "Brian", "Dan", "Eli", "Franklin"]
players.swapAt(1, three) print(players) // > ["Anna", "Brian", "Craig", "Donna", "Dan", "Eli", "Franklin"]
players.sort() impress(players) // > ["Anna", "Brian", "Craig", "Dan", "Donna", "Eli", "Franklin"]
Iterating through an array
Information technology'south getting belatedly, so the players decide to stop for the night and proceed tomorrow. In the meantime, y'all'll proceed their scores in a split array. You lot'll investigate a meliorate approach for this when you learn well-nigh dictionaries, just for now you can continue to use arrays:
let scores = [2, 2, eight, 6, ane, ii, ane]
for histrion in players { print(player) } // > Anna // > Brian // > Craig // > Dan // > Donna // > Eli // > Franklin
for (index, player) in players.enumerated() { print("\(index + 1). \(player)") } // > i. Anna // > 2. Brian // > 3. Craig // > 4. Dan // > 5. Donna // > 6. Eli // > 7. Franklin
func sumOfElements(in array: [Int]) -> Int { var sum = 0 for number in assortment { sum += number } return sum }
print(sumOfElements(in: scores)) // > 22
Mini-exercise
Write a for-in
loop that prints the players' names and scores.
Running time for array operations
Arrays are stored as a contiguous cake in memory. That means if you have x elements in an array, the ten values are all stored one adjacent to the other. With that in heed, here's the performance cost of diverse assortment operations:
Dictionaries
A lexicon is an unordered collection of pairs, where each pair comprises a key and a value.
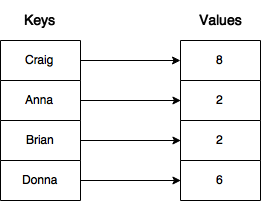
Creating dictionaries
The easiest way to create a dictionary is by using a dictionary literal. This is a list of key-value pairs separated past commas, enclosed in foursquare brackets.
var namesAndScores = ["Anna": 2, "Brian": 2, "Craig": viii, "Donna": half dozen] impress(namesAndScores) // > ["Craig": 8, "Anna": 2, "Donna": 6, "Brian": two]
namesAndScores = [:]
var pairs: [Cord: Int] = [:]
pairs.reserveCapacity(20)
Accessing values
As with arrays, there are several means to access dictionary values.
Using subscripting
Dictionaries support subscripting to access values. Unlike arrays, y'all don't admission a value past its index but rather past its key. For instance, if you want to go Anna's score, you would type:
namesAndScores = ["Anna": 2, "Brian": ii, "Craig": viii, "Donna": 6] // Restore the values print(namesAndScores["Anna"]!) // 2
namesAndScores["Greg"] // nil
Using properties and methods
Dictionaries, similar arrays, accommodate to Swift's Collection
protocol. Because of that, they share many of the same properties. For example, both arrays and dictionaries have isEmpty
and count
properties:
namesAndScores.isEmpty // simulated namesAndScores.count // 4
Modifying dictionaries
It's easy enough to create dictionaries and admission their contents — but what almost modifying them?
Adding pairs
Bob wants to bring together the game.
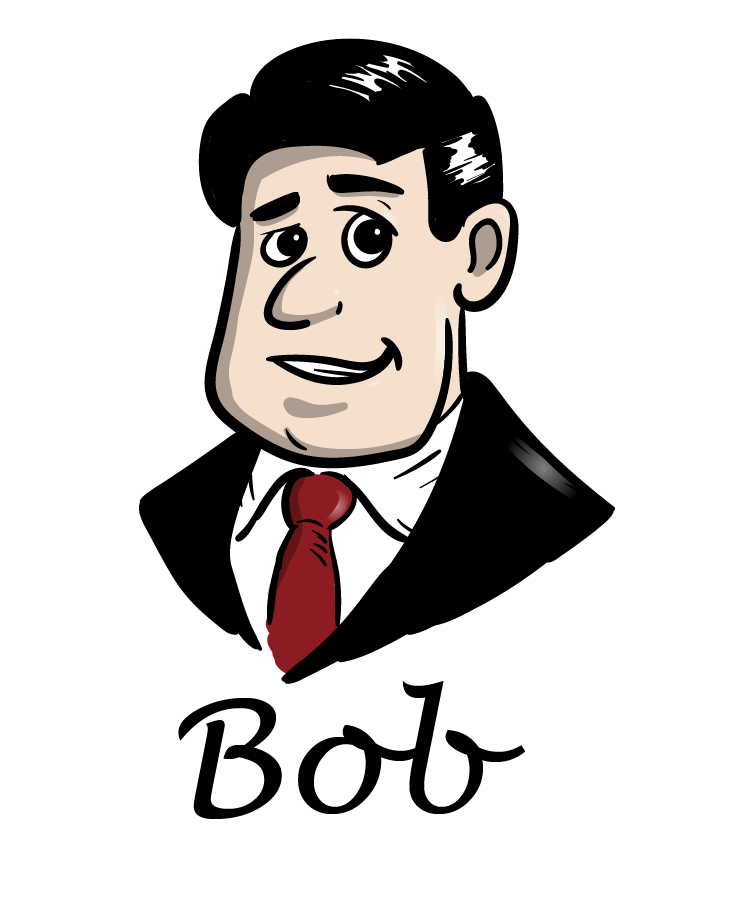
var bobData = [ "proper noun": "Bob", "profession": "Card Actor", "country": "USA" ]
bobData.updateValue("CA", forKey: "land")
bobData["city"] = "San Francisco"
Mini-practice
Write a role that prints a given player's metropolis and country.
Updating values
It appears that in the past, Bob was caught adulterous when playing cards. He's not just a professional — he's a carte shark! He asks you to modify his name and profession and then no one will recognize him.
bobData.updateValue("Bobby", forKey: "name") // Bob
bobData["profession"] = "Mailman"
Removing pairs
Bob — er, sorry — Bobby, all the same doesn't feel safe, and he wants you lot to remove all data about his whereabouts:
bobData.removeValue(forKey: "state")
bobData["city"] = zero
Iterating through dictionaries
The for-in
loop likewise works when you want to iterate over a dictionary. But since the items in a lexicon are pairs, y'all demand to use a tuple:
for (actor, score) in namesAndScores { impress("\(player) - \(score)") } // > Craig - eight // > Anna - 2 // > Donna - 6 // > Brian - 2
for histrion in namesAndScores.keys { print("\(role player), ", terminator: "") // no newline } impress("") // impress ane final newline // > Craig, Anna, Donna, Brian,
Running time for dictionary operations
In order to exist able to examine how dictionaries piece of work, you need to understand what hashing is and how information technology works. Hashing is the process of transforming a value — String
, Int
, Double
, Bool
, etc — to a numeric value, known as the hash value. This value can and so be used to quickly lookup the values in a hash table.
Sets
A set up is an unordered collection of unique values of the same type. This tin can be extremely useful when you desire to ensure that an item doesn't appear more than once in your collection, and when the order of your items isn't important.
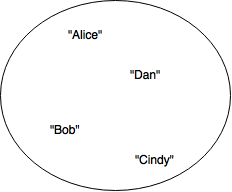
Creating sets
You can declare a set explicitly by writing Set
followed past the type inside angle brackets:
permit setOne: Set up<Int> = [i]
Set up literals
Sets don't accept their ain literals. You lot use array literals to create a ready with initial values. Consider this instance:
let someArray = [one, 2, iii, 1]
var explicitSet: Ready<Int> = [1, two, 3, 1]
var someSet = Set up([one, 2, iii, i])
print(someSet) // > [two, iii, 1] merely the social club is not defined
Accessing elements
Yous can utilise contains(_:)
to check for the being of a specific element:
print(someSet.contains(1)) // > true print(someSet.contains(4)) // > false
Calculation and removing elements
You lot can use insert(_:)
to add elements to a set. If the element already exists, the method does nothing.
someSet.insert(5)
let removedElement = someSet.remove(ane) print(removedElement!) // > ane
Running time for set operations
Sets have a very similar implementation to those of dictionaries, and they as well require the elements to be hashable. The running time of all the operations is identical to those of dictionaries.
Challenges
Earlier moving on, here are some challenges to test your knowledge of arrays, dictionaries and sets. It is best to try to solve them yourself, just solutions are available if you get stuck. These came with the download or are bachelor at the printed volume's source lawmaking link listed in the introduction.
Challenge 1: Which is valid
Which of the post-obit are valid statements?
1. permit array1 = [Int]() 2. permit array2 = [] three. let array3: [String] = []
let array4 = [1, 2, iii]
4. print(array4[0]) 5. print(array4[v]) six. array4[ane...2] vii. array4[0] = 4 viii. array4.append(4)
var array5 = [one, two, iii]
9. array5[0] = array5[one] 10. array5[0...one] = [four, five] xi. array5[0] = "Half dozen" 12. array5 += 6 13. for item in array5 { print(detail) }
Challenge 2: Remove the first number
Write a function that removes the kickoff occurrence of a given integer from an assortment of integers. This is the signature of the part:
func removingOnce(_ particular: Int, from array: [Int]) -> [Int]
Challenge three: Remove the numbers
Write a function that removes all occurrences of a given integer from an array of integers. This is the signature of the role:
func removing(_ item: Int, from array: [Int]) -> [Int]
Claiming 4: Reverse an assortment
Arrays have a reversed()
method that returns an assortment holding the same elements every bit the original assortment, in reverse order. Write a function that does the same thing, without using reversed()
. This is the signature of the function:
func reversed(_ array: [Int]) -> [Int]
Challenge 5: Render the middle
Write a part that returns the centre element of an assortment. When array size is fifty-fifty, render the first of the two middle elememnts.
func centre(_ array: [Int]) -> Int?
Challenge vi: Find the minimum and maximum
Write a function that calculates the minimum and maximum value in an array of integers. Calculate these values yourself; don't use the methods min
and max
. Return nil
if the given array is empty.
func minMax(of numbers: [Int]) -> (min: Int, max: Int)?
Challenge 7: Which is valid
Which of the post-obit are valid statements?
ane. let dict1: [Int, Int] = [:] 2. allow dict2 = [:] 3. allow dict3: [Int: Int] = [:]
let dict4 = ["One": 1, "Two": ii, "Three": 3]
4. dict4[i] five. dict4["One"] 6. dict4["Zilch"] = 0 seven. dict4[0] = "Nothing"
var dict5 = ["NY": "New York", "CA": "California"]
viii. dict5["NY"] ix. dict5["WA"] = "Washington" 10. dict5["CA"] = zilch
Challenge 8: Long names
Given a dictionary with 2-letter state codes as keys, and the full state names as values, write a function that prints all united states with names longer than eight characters. For instance, for the dictionary ["NY": "New York", "CA": "California"]
, the output would exist California
.
Challenge 9: Merge dictionaries
Write a part that combines 2 dictionaries into one. If a certain key appears in both dictionaries, ignore the pair from the get-go dictionary. This is the function's signature:
func merging(_ dict1: [String: String], with dict2: [Cord: String]) -> [String: Cord]
Challenge 10: Count the characters
Declare a function occurrencesOfCharacters
that calculates which characters occur in a string, as well as how often each of these characters occur. Return the event as a dictionary. This is the office signature:
func occurrencesOfCharacters(in text: String) -> [Character: Int]
Challenge 11: Unique values
Write a role that returns true
if all of the values of a dictionary are unique. Utilize a set to test uniqueness. This is the role signature:
func isInvertible(_ lexicon: [String: Int]) -> Bool
Claiming 12: Removing keys and setting values to nil
Given the dictionary:
var nameTitleLookup: [String: String?] = ["Mary": "Engineer", "Patrick": "Intern", "Ray": "Hacker"]
Central points
Sets
8. Collection Iteration with Closures half dozen. Optionals
How To Add Dictionary To Array In Swift,
Source: https://www.raywenderlich.com/books/swift-apprentice/v6.0/chapters/7-arrays-dictionaries-sets
Posted by: millswhimen.blogspot.com
0 Response to "How To Add Dictionary To Array In Swift"
Post a Comment