How To Add Into A List Python
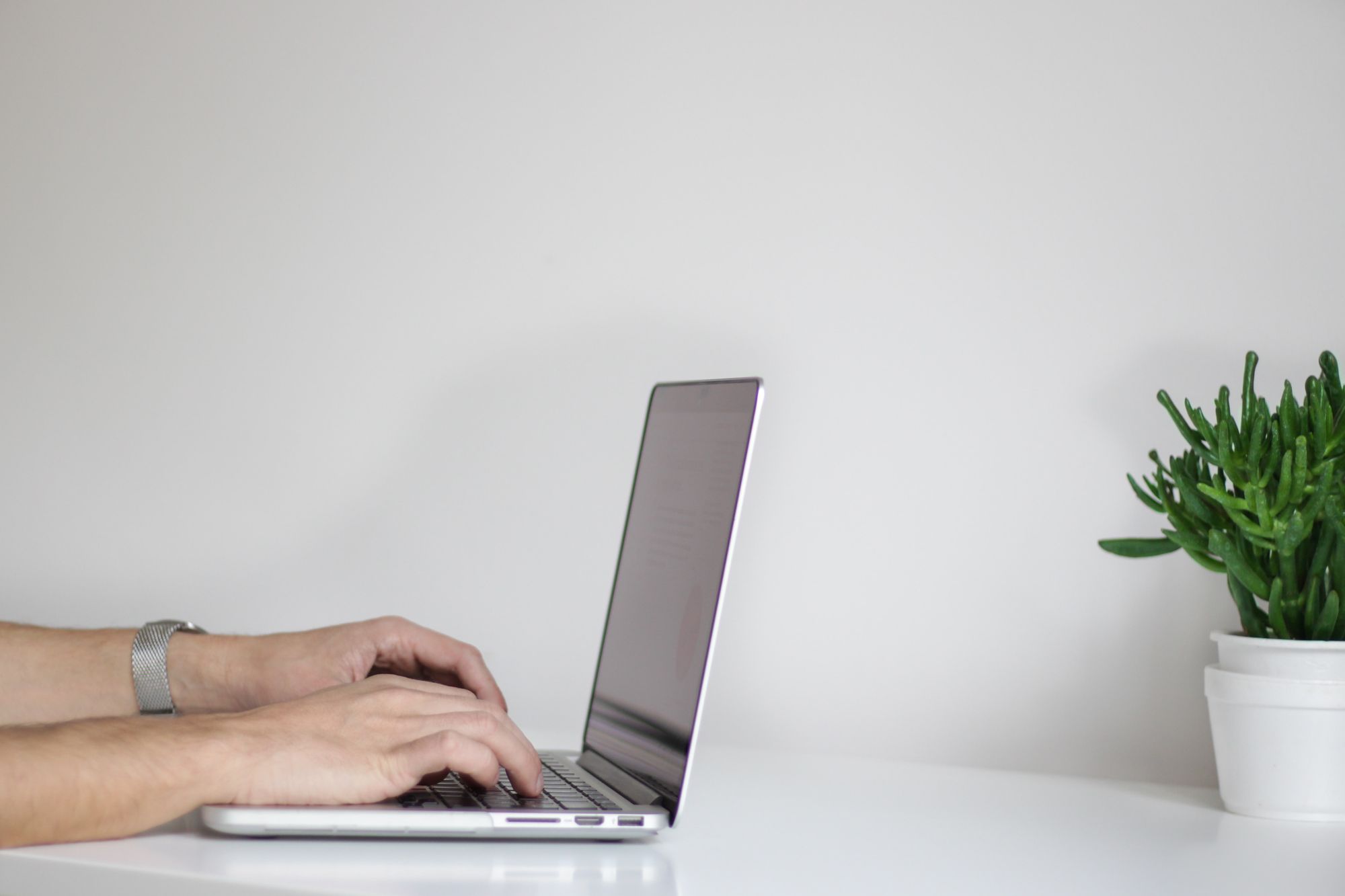
In this article, you'll learn most the .append()
method in Python. You'll also run into how .suspend()
differs from other methods used to add elements to lists.
Let's become started!
What are lists in Python? A definition for beginners
An array in programming is an ordered collection of items, and all items need to exist of the same data type.
However, unlike other programming languages, arrays aren't a built-in data structure in Python. Instead of traditional arrays, Python uses lists.
Lists are essentially dynamic arrays and are ane of the most mutual and powerful data structures in Python.
You tin can think of them every bit ordered containers. They shop and organize similar kind of related data together.
The elements stored in a list tin be of any data type.
There can exist lists of integers (whole numbers), lists of floats (floating point numbers), lists of strings (text), and lists of whatever other built-in Python data blazon.
Although it is possible for lists to hold items of only the same data type, they are more than flexible than traditional arrays. This means that there tin be a variety of unlike data types inside the same list.
Lists take 0 or more items, meaning in that location can besides be empty lists. Inside a listing in that location can also be duplicate values.
Values are separated past a comma and enclosed in foursquare brackets, []
.
How to create lists in Python
To create a new list, outset give the listing a proper noun. Then add the assignment operator(=
) and a pair of opening and closing square brackets. Inside the brackets add the values you want the listing to comprise.
#create a new list of names names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #impress the listing to the console impress(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny']
How lists are indexed in Python
Lists maintain an order for each item.
Each detail in the collection has an its ain alphabetize number, which you can apply to admission the item itself.
Indexes in Python (and every other mod programming linguistic communication) start at 0 and increment for every detail in the list.
For example, the list created before on had iv values:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"]
The starting time value in the listing, "Jimmy", has an index of 0.
The second value in the list, "Timmy", has an index of one.
The tertiary value in the list, "Kenny", has an index of 2.
The 4th value in the list, "Lenny", has an alphabetize of three.
To access an chemical element in the listing past its index number, first write the name of the list, then in foursquare brackets write the integer of the element's index.
For example, if you wanted to access the element that has an index of ii, you'd do:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] print(names[ii]) #output #Kenny
Lists in Python are mutable
In Python, when objects are mutable, it means that their values tin be changed once they've been created.
Lists are mutable objects, so you can update and change them after they accept been created.
Lists are also dynamic, meaning they can grow and shrink throughout the life of a program.
Items can be removed from an existing list, and new items tin can be added to an existing list.
At that place are built-in methods for both adding and removing items from lists.
For example, to add items, at that place are the .append()
, .insert()
and .extend()
methods.
To remove items, in that location are the .remove()
, .pop()
and .pop(index)
methods.
What does the .suspend()
method do?
The .append()
method adds an additional element to the end of an already existing list.
The general syntax looks something similar this:
list_name.append(item)
Let's interruption information technology down:
-
list_name
is the name you've given the list. -
.append()
is the list method for adding an item to the terminate oflist_name
. -
item
is the specified private item y'all want to add together.
When using .append()
, the original list gets modified. No new list gets created.
If you wanted to add an extra proper noun to the list created from before on, you lot would do the following:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #add together the name Dylan to the end of the list names.append("Dylan") print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny', 'Dylan']
What'southward the difference between the .suspend()
and .insert()
methods?
The departure between the two methods is that .append()
adds an item to the end of a list, whereas .insert()
inserts and particular in a specified position in the list.
As you lot saw in the previous department, .append()
will add the item y'all pass equally the argument to the function always to the end of the list.
If you don't desire to just add together items to the end of a list, you can specify the position yous want to add them with .insert()
.
The general syntax looks similar this:
list_name.insert(position,detail)
Let'southward pause information technology down:
-
list_name
is the name of the list. -
.insert()
is the listing method for inserting an detail in a listing. -
position
is the first argument to the method. It's ever an integer - specifically information technology'south the index number of the position where you lot want the new item to be placed. -
item
is the second argument to the method. Here you specify the new particular you want to add together to the list.
For example, say you had the post-obit list of programming languages:
programming_languages = ["JavaScript", "Coffee", "C++"] impress(programming_languages) #output #['JavaScript', 'Java', 'C++']
If you wanted to insert "Python" at the showtime of the list, every bit a new item to the list, you would use the .insert()
method and specify the position equally 0
. (Call up that the starting time value in a listing ever has an index of 0.)
programming_languages = ["JavaScript", "Java", "C++"] programming_languages.insert(0, "Python") print(programming_languages) #output #['Python', 'JavaScript', 'Java', 'C++']
If you instead had wanted "JavaScript" to exist the showtime item in the list, and and so add "Python" as the new item, you would specify the position as 1
:
programming_languages = ["JavaScript", "Java", "C++"] programming_languages.insert(1,"Python") print(programming_languages) #output #['JavaScript', 'Python', 'Java', 'C++']
The .insert()
method gives you a chip more than flexibility compared to the .append()
method which only adds a new item to the end of the listing.
What's the difference between the .append()
and .extend()
methods?
What if you desire to add more than than ane item to a list at in one case, instead of adding them one at a time?
You can use the .append()
method to add more than one particular to the end of a list.
Say you have ane listing that contains merely two programming languages:
programming_languages = ["JavaScript", "Java"] impress(programming_languages) #output #['JavaScript', 'Java']
Yous and then desire to add together two more languages, at the end of information technology.
In that instance, you lot pass a listing containing the two new values you want to add, as an argument to .append()
:
programming_languages = ["JavaScript", "Java"] #add 2 new items to the end of the list programming_languages.suspend(["Python","C++"]) impress(programming_languages) #output #['JavaScript', 'Java', ['Python', 'C++']]
If you have a closer look at the output from above, ['JavaScript', 'Java', ['Python', 'C++']]
, you'll see that a new list got added to the cease of the already existing listing.
So, .append()
adds a list inside of a listing.
Lists are objects, and when you use .suspend()
to add another list into a list, the new items volition be added as a single object (particular).
Say you already had two lists, like and then:
names = ["Jimmy", "Timmy"] more_names = ["Kenny", "Lenny"]
What if wanted to combine the contents of both lists into one, by adding the contents of more_names
to names
?
When the .suspend()
method is used for this purpose, another list gets created inside of names
:
names = ["Jimmy", "Timmy"] more_names = ["Kenny", "Lenny"] #add together contents of more_names to names names.append(more_names) impress(names) #output #['Jimmy', 'Timmy', ['Kenny', 'Lenny']]
Then, .append()
adds the new elements as another list, by appending the object to the end.
To actually concatenate (add) lists together, and combine all items from i list to another, y'all need to employ the .extend()
method.
The general syntax looks like this:
list_name.extend(iterable/other_list_name)
Allow's interruption information technology down:
-
list_name
is the proper noun of ane of the lists. -
.extend()
is the method for adding all contents of one list to another. -
iterable
can be any iterable such as another list, for example,another_list_name
. In that case,another_list_name
is a list that will be concatenated withlist_name
, and its contents will be added one past one to the stop oflist_name
, as separate items.
Then, taking the instance from earlier on, when .append()
is replaced with .extend()
, the output will look like this:
names = ["Jimmy", "Timmy"] more_names = ["Kenny", "Lenny"] names.extend(more_names) print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny']
When we used .extend()
, the names
list got extended and its length increased past two.
The way .extend()
works is that it takes a list (or other iterable) as an statement, iterates over each chemical element, and then each element in the iterable gets added to the list.
There is another divergence between .suspend()
and .extend()
.
When yous want to add a string, as seen earlier, .suspend()
adds the whole, single item to the end of the listing:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #add the name Dylan to the finish of the list names.append("Dylan") print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny', 'Dylan']
If you used .extend()
instead to add a string to the stop of a list ,each character in the string would be added every bit an private item to the list.
This is because strings are an iterable, and .extend()
iterates over the iterable argument passed to information technology.
Then, the example from above would await like this:
names = ["Jimmy", "Timmy", "Kenny", "Lenny"] #pass a string(iterable) to .extend() names.extend("Dylan") print(names) #output #['Jimmy', 'Timmy', 'Kenny', 'Lenny', 'D', 'y', 'l', 'a', 'north']
Conclusion
To sum up, the .append()
method is used for calculation an detail to the cease of an existing list, without creating a new listing.
When information technology is used for adding a list to another listing, it creates a list within a list.
If you want to acquire more nigh Python, check out freeCodeCamp'due south Python Certification. You lot'll outset learning in an interacitve and beginner-friendly fashion. You'll also build v projects at the end to put into do what you learned.
Thanks for reading and happy coding!
Learn to code for free. freeCodeCamp's open up source curriculum has helped more than 40,000 people get jobs every bit developers. Get started
How To Add Into A List Python,
Source: https://www.freecodecamp.org/news/append-in-python-how-to-append-to-a-list-or-an-array/
Posted by: millswhimen.blogspot.com
0 Response to "How To Add Into A List Python"
Post a Comment